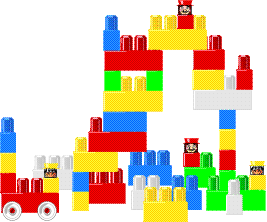
Essential Perl
Perl stands for "Practical Extraction and Report Language." It accounts for over 50% of the CGIs written on the Web (World Wide Web Programming, Tittel, Gaither, Hassinger and Erwin,
IDGBooks, 1995) and can be used on Unix, Windows 95 and NT. I strongly suggest that you read the Learning Perl book, if you are interested in learning Perl in more details. You can also find a Perl Manual
here. It has also been ported to other systems like Macintosh, OS/2, etc. Read the Wikipedia Wiki Perl page for more information on Perl 5 versions and also Perl 6.
Perl 5 is installed at the UB open labs under Windows . You do NOT need to install it at the labs. At the MIS Lab both Windows and Linux have Perl 5 installed. Remotely, download the current version for Windows from ActiveWare. If you have Linux installed home it already has Perl 5 installed as part of most distributions.
Perl syntax overview
Introduction
Comments in Perl are marked with the # (number) sign and run to the end of the line. All
statement lines end with ; (semicolon). There are three types of variables in Perl -- scalar ($),
array (@) and associative array (%). Perl supports a variety of control structures -- block statements,
if/else, unless, while/until, for, foreach (no goto is really supported). Perl provides easy I/O
statements -- STDIN, STDOUT, print/printf, open/close (filehandles), die, format, as well as
directory access. Finally, functions/subroutines are also easy to create and use. A variety of Perl
libraries are available for simplifying many of the Web tasks.
Variables
- Scalar variables.
Scalar variables can be numeric or strings and start with a $ sign, as in
$name. They contain one single value like 34.5 or "Have a nice day!". Please note that strings
are enclosed in quotes. There are two types of quotes used 'single' and "double" . I
recommend that beginners use double-quotes for they allow interpolation (translation) of
variable-names and allows \ (backslash) to specify control characters. For example Print "This
is my $name \n"; will print the quoted text with the value of $name replaced by its value,
followed by a new line. See example 1
Array variables. Array variables are an ordered list of scalar data and start with @ sign, as in
@lots. Each element is an individual variable with a scalar value, separated by commas, as in
@a = ($a, 17, "nice day"); Subscripts start from 0 (zero), so $a [1] has the value 17. Please
note that $a and $a[1] are not the same variables. For $a [1] is the scalar value of the @a array
variable first element, while $a is a simple scalar variable. See example 2
Associative arrays. Associative array variables are an unordered list of pairs of scalar keys
and values, separated by commas and start with a % sign, as in %a. As in arrays we use
associative arrays through their scalar values, like $a{age} = 34; Meaning that we are
assigning the value 34 to be paired with the key age (we may also be creating the key in this
statement). Please note that we are using curly braces { instead of brackets [ to identify each
element of the list of the associative array. See example 3
Some scalar operators. There are too many operators in Perl for me to summarize here. The
traditional +, -, *, /, **, <,>, <=, >=. ==. != operators work for numeric variables, while string
variables use lt, gt, le, ge, eq, ne for comparisons, and can be concatenated with . (dot). The
logical end is represented by && , the logical or by | |, and the logical not by ! ~ .
Autoincrement and autodecrement are represented by ++ and -- , respectively, like in ++$a;
meaning $a=$a+1; Binary assignment operators are also supported like in $a+=5; and $a*=3;
(adds 5 to $a and assigns result to $a, multiply $a by 3 and assigns the result to $a). See example 4
Control structures
- Block statements
. A statement block is a sequence of statements enclose in curly braces { .... }
, as in { ++a$; $b/$a; } It can go wherever a statement goes and have the value of the last
statement and is true if not zero or empty, false otherwise.
If-else, if-elsif-else. It follows the general pattern: if (expression) { true statements } elsif
(expression) { truelsif statements) } else { falsestatements } Please note that statements end in
semi-colon but not the if control structure, it starts with curly braces and ends with curly
braces (required). See example 5
While/Until. These are loop control structures used respectively for a true expression
continue, or a false expression stop. Patterns: while (expression) { statements) } and until
(expression) { statements}
For/Foreach These are loop control expressions with a control variable or expression.
Patterns: for (initial-expression;test-expression;increment-expression) { statements } and
foreach $i (@somelist) { statements}. You can omit the control scalar variable and Perl will
default to the $_ variable as a scratch variable (this is also true in many other circumstances). See example 6
I/O Statements
- <STDIN>
In a scalar context it gives the next line of the standard input device, or undef if
there are no more lines. In an array context gives all lines as a list. So $a=<STDIN>; reads
one line in $a, while @a=<STDIN> reads all lines into @a. A line in Perl always includes a \n
(newline) at the end, and we have to remove it before processing the line value. We use
chop($var) to remove the \n. A common pattern to read, remove the newline and process
input is as follows: while (<STDIN>) { chop; statements using $_ and other variables } .
Please note that we are using while to create a loop controlled by the default $_= <STDIN>
scratch variable, and also using the $_ by default in chop, as if we have written chop($_). See example 7
Print/Printf. Print the output to either STDOUT -- the standard output device, or to a file
handle (we will see filehandles below in the Open statement. Pattern: print "text $var \n";
Simple format is also provided by printf. Pattern: printf "format", v1, v2, vn , where the
format is comprised by %xs, %yd and %z.yf, separated by commas and followed by \n. This
means that you will have a variable with x string characters, with y numeric decimal integer
number, and with z decimal digits followed by y digits after the decimal point for floating-point numbers.
Open/Close. Open selects a file to be used as input, output or input/output, instead of STDIN
and STDOUT. Perl calls "filehandles" the logical names of the files opened. Patterns: open
(filehandle, "fname"); open (filehandle, ">fname"); and open (filehandle, ">>fname");
respectively, open for input, output and input/output -- append. The "fname" is the external,
system, name of the file, while filehandle is the internal, logical, name of the file. Example:
open (DATA, "file.txt"); and use <DATA> with angled brackets to represent I/O like in
<STDIN>. Please note that it is recommended that filehandles be capitalized. Also, Pattern:
close (filehandle);
Die. Allow testing if the open was successful, meaning if you could open the file. It is used
with open. Pattern: open (filehandle, "fname") | | die " Sorry I couldn't open the file\n" See for files example 8
Functions/Subroutines
- Defining
. Subroutines are defined using the pattern: sub subname { statements } . They can be
placed anywhere in the script, but usually are placed at the end. Be aware that the return value
of a sub is the last expression evaluated, literally.
Invoking. You call or invoke a sub by putting an ampersand & before its name. Pattern:
&subname . A sub can invoke, another sub, that in turn can invoke another, etc. There is no
limit (other than efficiency) for the levels of invocation. The return value of a sub can be
assigned to variables.
Arguments. A sub can be invoked with arguments. Pattern: &subname (var1, var2,varn) .
The list of arguments is automatically assigned to @_ , and you can refer to the arguments in
the sub as $_[i], remember that subscripts start from zero, so the first argument is $_[0], the
second $ _[1], etc. See example 9
Including. To include a sub available in a library in your Perl directory (another Perl script),
use the Pattern: require "scriptname.pl". Please note that subs you have in your own script you
invoke like described above in item 2. You include a script that you have in your Perl lib sub-directory. Once you include, you can use all functions that the script supports. This is how we
use specific subs created to support the Web. Of course, some times you can just modify an
existing script and make it yours if it is freeware and the author allows you to do so.
I also included two other examples here.
This page is maintained by Al Bento
who can be reached at abento@ubalt.edu. This page was last updated on August 5, 2017, twenty years after it was created. Although we will attempt to keep this information accurate, we can not guarantee the accuracy of the information provided.